12. Why did we learn the plubming commands?#
Important
If you miss class or lab for any reason you are responsible for making it up per the instructions in the syllabus. You do not need to notify me why you are missing class and I do not judge your reasons for missing class for short absences. For longer absences you may email me to make a plan.
For lab makeup this includes attending office hours for the checkout step.
For class makeup this includes an alternative path to your experience badge. Note that in class actitivies may build, so it is important to review the notes ASAP so that you are ready to participate in the next class you attend.
You will not typically use them on a day to day basis, but they are a good way to see what happens at the interim steps and make sure that you have the right understanding of what git does.
A correct understanding is essential for using more advanced features
While there is of course some content that we want you to know after this course, my goal is also to teach you process, by modeling it.
No one will every know all of the things but you can be fast or slow at finding answers.
And you can find correct answers, incorrect answers, or looks-okay-but-you-will-regret-this-later answers.
My goal is that you get good at quickly finding correct answers.
Large language models will not do that for you.
Note I checked and confirmed that some of the assignments will be answered incorrectly by LLMs because they are about very specific details that most people who write blogs and code excerpts on stack overflow etc do incorrectly.
12.1. Today’s Questions#
What are references?
How can can I release and share my code?
How else can git help me?
12.2. What does git status do?#
compares the working directory to the current state of the active branch
we cansee the working directory with:
ls
we can see the active branch in the
HEAD
filewhat is its status?
Recall that we:
crated a blob objct directly
creataed a file
hashed the file, to create its blob object
added the file to the index
wrote the tree
created a commit
Let’s use inspection to review where our repo is left off from last week.
git status
On branch main
No commits yet
Changes to be committed:
(use "git rm --cached <file>..." to unstage)
new file: test.txt
Changes not staged for commit:
(use "git add <file>..." to update what will be committed)
(use "git restore <file>..." to discard changes in working directory)
modified: test.txt
We see that git status still thinks the file is only staged, but not committed.
From this we learn that index is not emptied when we create a commit.
We see that the file is both staged and has changes not staged because we edited the file after we had staged it.
This reminds us that the staging area is manual, like all git operations. git does not run continously, which makes it faster and less resource intensive.
We also note that this says we are on the main branch , and that we have no commits yet. Let’s try to understand why it says we have no commits when we thougth we made one
12.3. Branches are references#
branches are not git objects, they are references.
We can see that by where they are stored.
How can we inspect to see where references are stored?
using ls and/or find
12.3.1. Why is our commit missing?#
We can see our list of object with find
find .git/objects/ -type f
.git/objects//0c/1e7391ca4e59584f8b773ecdbbb9467eba1547
.git/objects//d6/70460b4b4aece5915caf5c68d12f560a9fe3e4
.git/objects//d8/329fc1cc938780ffdd9f94e0d364e0ea74f579
.git/objects//e3/ba10cb02de504d4f48b9af4934ddcc4d0be3df
.git/objects//83/baae61804e65cc73a7201a7252750c76066a30
Since we made most of these objects as not commits, the hashes are mostly shared, but one of the hashes is unique so we worked together to identify that one.
Then for that unique hash, we confirmed it was a commit using git cat-file
to view its type
git cat-file -t e3ba
commit
as expected
Now let’s look at what the HEAD
pointer says to try to understand why it does not see that commit, since we know that git status works from HEAD
cat .git/HEAD
ref: refs/heads/main
this is consistent with the git status
said, so this is not yet explaining
now we can check what the branch points to
cat .git/refs/heads/main
as expected when the tab complete did not work
cat: .git/refs/heads/main: No such file or directory
it has not even made a file to track what commit the main branch points to.
Important
git creates updates the file for the branch each time you add a commit with git commit
, the first time git commit
is run it also creates the file
If we look in the folder, we can see what is in there
ls .git/refs/heads/
but it is empty
12.3.2. Updating a branch manually#
The branch file is named as the branch (here main
) and stored in the .git/refs/heads/
folder and contains the full hash of the commit that branch is pointing to.
Since we made the commit manually, we need to move the branch manually too. We will use echo, by copyting the full hash from above (I copied the part after the last /
in the list of objects above and then typed the e3
before pasting).
Mine looks like:
echo e3ba10cb02de504d4f48b9af4934ddcc4d0be3df > .git/refs/heads/main
but your commit hash will be different than mine.
Now we check with git again:
git status
On branch main
Changes not staged for commit:
(use "git add <file>..." to update what will be committed)
(use "git restore <file>..." to discard changes in working directory)
modified: test.txt
no changes added to commit (use "git add" and/or "git commit -a")
and now we can see that it no longer sees the staged file and does see our commit.
So we now have HEAD-> main and main -> our commit -> tree –> blob.
12.5. Debugging with git#
git will help you do a binary search to find what commit introduced a bug.
we have to tell it to start, then tell it that the current commit is bad, then tell it the last good commit (or a reference to it)
To do this we need to have to tell it what commit was good, this is one of the reasons that using tags can be helpful
there are multiple ways to refer to a point in the history
First let’s choose a point to use as our last good commit by scanning the previous commits.
git log
commit 0d6ef1d3db0729088d515b35b588f39af5b770fd (HEAD -> main, tag: v1, origin/main, origin/HEAD)
Merge: 9f39946 e3b192a
Author: Sarah Brown <brownsarahm@uri.edu>
Date: Thu Feb 8 13:43:19 2024 -0500
Merge pull request #6 from compsys-progtools/organization
first pass organizing
commit e3b192aa0cd490226e8adcd81d3d0b95adb5676b (origin/organization, organization)
Author: Sarah M Brown <brownsarahm@uri.edu>
Date: Tue Feb 6 13:41:53 2024 -0500
oranizizng
commit 260c9c309922970f80bfa2c93cc23bcfbb962740
Author: Sarah M Brown <brownsarahm@uri.edu>
Date: Tue Feb 6 13:06:20 2024 -0500
describe the files
commit 29ffc88519103085ed3a2ab01cffb3c99d70fc6a
Author: Sarah M Brown <brownsarahm@uri.edu>
Date: Tue Feb 6 12:59:20 2024 -0500
add to readme
commit 9f399466ad6a1ad572e104209f4469eb8cd48516
Merge: 1e2a45f fca59e8
Author: Sarah Brown <brownsarahm@uri.edu>
Date: Tue Feb 6 12:43:18 2024 -0500
Merge pull request #5 from compsys-progtools/organizing_ac
2/6 in class activity
commit fca59e8cca05bb0861f9348a40fe8300b3d55637 (origin/organizing_ac)
Author: Sarah M Brown <brownsarahm@uri.edu>
Date: Tue Feb 6 11:21:21 2024 -0500
add files for organizing
commit 1e2a45fbca5ce7bf775827f5f4dbe23b6561cff4 (my_branch_cehckoutb, my_branch)
Merge: faef6af 81c6f18
Author: Sarah Brown <brownsarahm@uri.edu>
Date: Thu Feb 1 12:51:17 2024 -0500
Merge pull request #4 from compsys-progtools/2-create-an-about-file
create about file close s #2
commit 81c6f187f146caaaf43d97bc1bb8ed237142f4c3 (origin/2-create-an-about-file, 2-create-an-about-file)
Author: Sarah M Brown <brownsarahm@uri.edu>
Date: Tue Jan 30 13:33:54 2024 -0500
create about file close s #2
:
2/6 in class activity
commit fca59e8cca05bb0861f9348a40fe8300b3d55637 (origin/organizing_ac)
Author: Sarah M Brown <brownsarahm@uri.edu>
Date: Tue Feb 6 11:21:21 2024 -0500
add files for organizing
Let’s choose the commit where we left the create-an-about
branch.
This log shows history like this
We will start git bisect
first, this is our search for the “bad commit”
git bisect start
status: waiting for both good and bad commits
it tells us its progress at each step and what we need to do next, tell it the good and bad commits
Now, lets tell it that the current commit is bad, this reprsents that we just rcevied the bug report.
git bisect bad
status: waiting for good commit(s), bad commit known
now we get the updated status, so now it has labeled the last commit on the main branch as bad
next we tell it what was the last known, not necessarily the last, good commit. We’ll use the about branch commit. This would represent a case where maybe we switched to to that branch locally, ran a test for the the new bug and it passed.
my commit was 81c6
, yours will be different
git bisect good 81c6
Bisecting: 3 revisions left to test after this (roughly 2 steps)
[9f399466ad6a1ad572e104209f4469eb8cd48516] Merge pull request #5 from compsys-progtools/organizing_ac
It tells us where it checked out
We wil label this commit as also bad
git bisect bad
Now git labels all of the commits between the good commit and bad commit as bad:
and it checks out the next commit we need to look at:
Bisecting: 0 revisions left to test after this (roughly 1 step)
[fca59e8cca05bb0861f9348a40fe8300b3d55637] add files for organizing
We will label this as good:
git bisect good
Now git tells us the first bad commit, and a bunch of details about that first bad commit:
9f399466ad6a1ad572e104209f4469eb8cd48516 is the first bad commit
commit 9f399466ad6a1ad572e104209f4469eb8cd48516
Merge: 1e2a45f fca59e8
Author: Sarah Brown <brownsarahm@uri.edu>
Date: Tue Feb 6 12:43:18 2024 -0500
Merge pull request #5 from compsys-progtools/organizing_ac
2/6 in class activity
API.md | 1 +
CONTRIBUTING.md | 1 +
LICENSE.md | 1 +
_config.yml | 1 +
_toc.yml | 1 +
abstract_base_class.py | 1 +
alternative_classes.py | 1 +
example.md | 1 +
helper_functions.py | 1 +
important_classes.py | 1 +
philosophy.md | 1 +
scratch.ipynb | 1 +
setup.py | 1 +
test_abc.py | 1 +
tests_alt.py | 1 +
tests_helpers.py | 1 +
tests_imp.py | 1 +
17 files changed, 17 insertions(+)
create mode 100644 API.md
create mode 100644 CONTRIBUTING.md
create mode 100644 LICENSE.md
create mode 100644 _config.yml
create mode 100644 _toc.yml
create mode 100644 abstract_base_class.py
create mode 100644 alternative_classes.py
create mode 100644 example.md
create mode 100644 helper_functions.py
create mode 100644 important_classes.py
create mode 100644 philosophy.md
create mode 100644 scratch.ipynb
create mode 100644 setup.py
create mode 100644 test_abc.py
create mode 100644 tests_alt.py
create mode 100644 tests_helpers.py
create mode 100644 tests_imp.py
Note our final labeling of commits is:
We did not need to inspect the merge commit because git could tell it was only a merge commit and did not add any changes to files; if it had been a merge conflict resovled with the merge commit, we would have had to check it.
Here we can then inspect what changes were made and figure out how to fix the bug.
Let’s dig a bit futher into what the current status is.
git status
HEAD detached at fca59e8
You are currently bisecting, started from branch 'main'.
(use "git bisect reset" to get back to the original branch)
Untracked files:
(use "git add <file>..." to include in what will be committed)
.secret
my_sectrest/
nothing added to commit but untracked files present (use "git add" to track)
This is new, we have checked out a commit, instead of a branch so we have a detached head
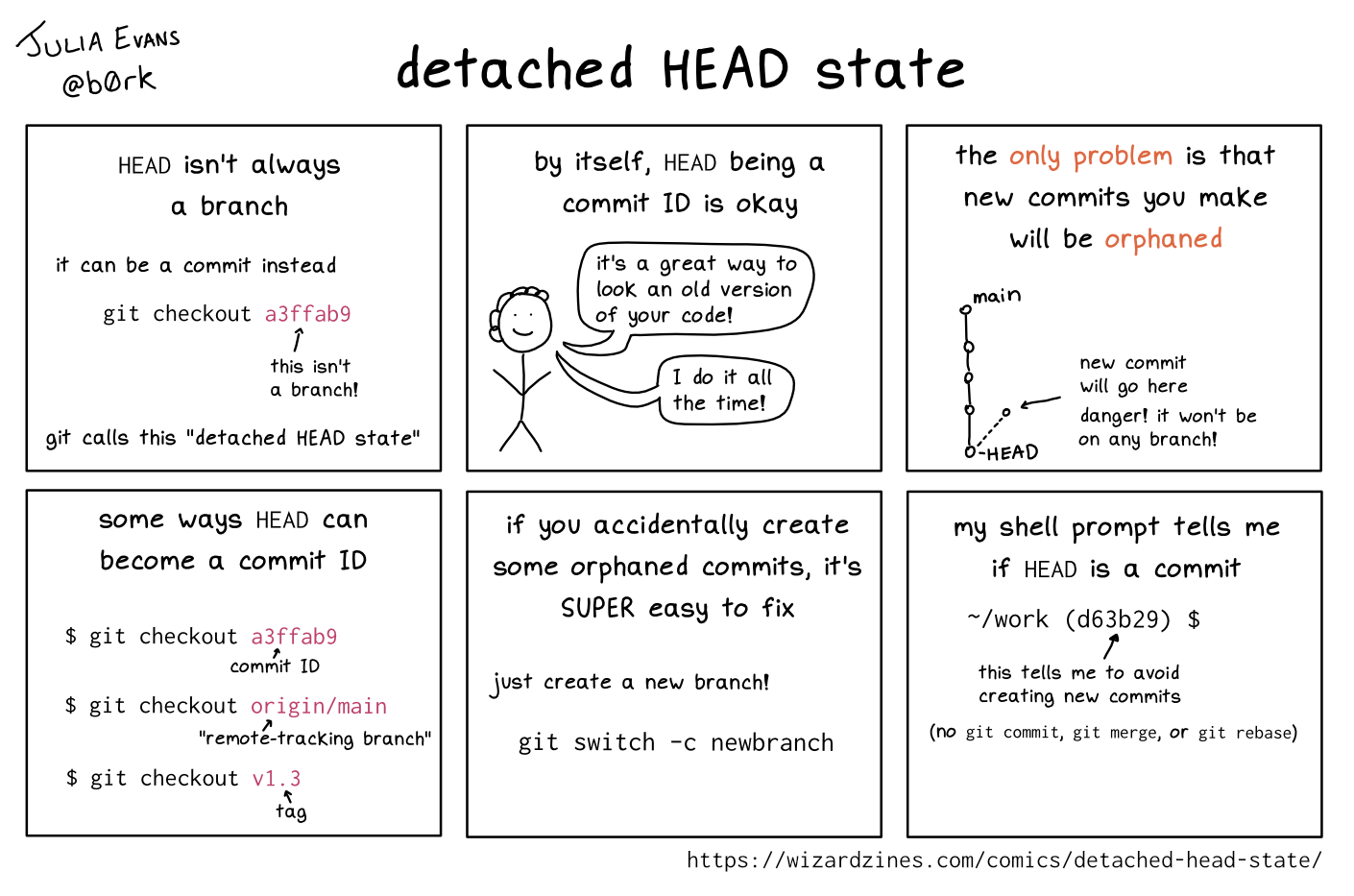
Fig. 12.1 A comic from wizard zines that has detailed alt text at the “Read the transript” link on the source page.#
We also see some untracked files. This is because at the tip of the main branch, where we were previously, these files existed and were listed in the .gitignore
We can see more about what bisect did by looking in the .git directory
ls .git/
BISECT_ANCESTORS_OK FETCH_HEAD index
BISECT_EXPECTED_REV HEAD info
BISECT_LOG ORIG_HEAD logs
BISECT_NAMES REBASE_HEAD objects
BISECT_START config packed-refs
BISECT_TERMS description refs
COMMIT_EDITMSG hooks
it created files for all of the steps in the active bisect
Now we go back to whre we were before the biset
git bisect reset
Previous HEAD position was fca59e8 add files for organizing
Switched to branch 'main'
Your branch is up to date with 'origin/main'.
in this case, main
git status
On branch main
Your branch is up to date with 'origin/main'.
nothing to commit, working tree clean
here we see the gitignroe is back
12.6. Any commit can be checked out#
We can also check out the error prone commit direct if we need to go back to fix again.
git checkout fca59
Note: switching to 'fca59'.
You are in 'detached HEAD' state. You can look around, make experimental
changes and commit them, and you can discard any commits you make in this
state without impacting any branches by switching back to a branch.
If you want to create a new branch to retain commits you create, you may
do so (now or later) by using -c with the switch command. Example:
git switch -c <new-branch-name>
Or undo this operation with:
git switch -
Turn off this advice by setting config variable advice.detachedHead to false
HEAD is now at fca59e8 add files for organizing
This way git gives us advice on how to handle our detached head.
12.7. Annotated commits create objects#
Maybe, we do not know how to fix this , but we want to make the commit easy for a colleague to find.
git tag -a -m 'introduced imaginary bug' v0.1
this time we will use -a
to make an annotated tag and pass the message for is with -m
We can
ls .git/refs/tags/
v0.1 v1
we see it created a file like before
So we can look at the file again
cat .git/refs/tags/v0.1
6b459a1dcf4e2a0ecec1ec41b03d60af4043e9a3
but this time the file points to a different object, not the commit.
We can inspect this object with git cat-file
:
git cat-file -t 6b45
tag
it is a new type: tag
and check its content
git cat-file -p 6b45
object fca59e8cca05bb0861f9348a40fe8300b3d55637
type commit
tag v0.1
tagger Sarah M Brown <brownsarahm@uri.edu> 1709663041 -0500
introduced imaginary bug
This is a lot like the content of a commit, but instead of pointing to a tree it points to the a commit.
12.8. How can git help with work in Progress?#
What if you have worked on something part way, but not finished it, so you do not want to make a commit yet, but you need to switch and work on something else?
Let’s go back to main
git checkout main
Previous HEAD position was fca59e8 add files for organizing
Switched to branch 'main'
Your branch is up to date with 'origin/main'.
and create some work in progress:
echo 'not done yet'>>example.md
we will look at our file
cat example.md
myst notebook example of using the code for doc websitenot done yet
and check in with git
git status
On branch main
Your branch is up to date with 'origin/main'.
Changes not staged for commit:
(use "git add <file>..." to update what will be committed)
(use "git restore <file>..." to discard changes in working directory)
modified: example.md
no changes added to commit (use "git add" and/or "git commit -a")
Now lets consider that we have some other work we need to do. We also do not want to lose this work, or make a commit for it yet. What can we do?
This is what the git “stash” is for. Stash is a first
git stash
stores temporary changes without making a commit so that we can come back to them.
git stash
Saved working directory and index state WIP on main: 0d6ef1d Merge pull request #6 from compsys-progtools/organization
We can see the impact of this like :
git status
On branch main
Your branch is up to date with 'origin/main'.
nothing to commit, working tree clean
Then we can do something else
echo "important " >> about.md
check in again
git status
On branch main
Your branch is up to date with 'origin/main'.
Changes not staged for commit:
(use "git add <file>..." to update what will be committed)
(use "git restore <file>..." to discard changes in working directory)
modified: about.md
no changes added to commit (use "git add" and/or "git commit -a")
make a new branch
git checkout -b important_about
Switched to a new branch 'important_about'
and add
git add .
we’ll check agian
git status
On branch important_about
Changes to be committed:
(use "git restore --staged <file>..." to unstage)
modified: about.md
our stashed content is still not interfering
and then commit this
git commit -m 'add about improtatn fact'
[important_about c3bee12] add about improtatn fact
1 file changed, 1 insertion(+)
now we can go back to main
git checkout main
Switched to branch 'main'
Your branch is up to date with 'origin/main'.
we can apply our changes with git stash apply
git stash apply
On branch main
Your branch is up to date with 'origin/main'.
Changes not staged for commit:
(use "git add <file>..." to update what will be committed)
(use "git restore <file>..." to discard changes in working directory)
modified: example.md
no changes added to commit (use "git add" and/or "git commit -a")
and we get them back
git checkout important_about
M example.md
Switched to branch 'important_about'
git status
On branch important_about
Changes not staged for commit:
(use "git add <file>..." to update what will be committed)
(use "git restore <file>..." to discard changes in working directory)
modified: example.md
no changes added to commit (use "git add" and/or "git commit -a")
git add .
git stash
Saved working directory and index state WIP on important_about: c3bee12 add about improtatn fact
git stash list
stash@{0}: WIP on important_about: c3bee12 add about improtatn fact
stash@{1}: WIP on main: 0d6ef1d Merge pull request #6 from compsys-progtools/organization
git status
On branch important_about
nothing to commit, working tree clean
git stash pop
On branch important_about
Changes not staged for commit:
(use "git add <file>..." to update what will be committed)
(use "git restore <file>..." to discard changes in working directory)
modified: example.md
no changes added to commit (use "git add" and/or "git commit -a")
Dropped refs/stash@{0} (4862b97ea83e0ddf04ca2344f33df90b1a78feb5)
git stash list
stash@{0}: WIP on main: 0d6ef1d Merge pull request #6 from compsys-progtools/organization
git add .
git status
On branch important_about
Changes to be committed:
(use "git restore --staged <file>..." to unstage)
modified: example.md
git checkout main
M example.md
Switched to branch 'main'
Your branch is up to date with 'origin/main'.
git status
On branch main
Your branch is up to date with 'origin/main'.
Changes to be committed:
(use "git restore --staged <file>..." to unstage)
modified: example.md
## Prepare for Next Class
```{include} ../_prepare/2024-03-07.md
12.9. Badges#
Use git bisect to find the first bad commit in the toy bug repo, save the command history and the bad commit hash to git_debug.md
Create tagtypeexplore.md with the template below. Determine how many of the tags in the course website are annotated vs lightweight using. (You may need to use
git pull --tags
in your clone of the course website)
# Tags
<!-- short defintion/description in your own words of what a tag is and what it is for -->
## Inspecting tags
Course website tags by type:
- annoted:
- lightweight:
Practice using git to fix problem using a git command from the patching or debugging section of the docs. Create a log of what you did using the history or git log into a file git_debug_story.md. If you have a project in another class or another badge in this class that causes you to use one of these features in a real scenario, that can count. If not, for example, you could create a “bug” and then use bisect to find it.
Create tagtypes.md with the template below. Include an experiment that shows which if either type of tag creates a new git object. There are two types, try creating one of each a lightwight tag (provide only the tag name- what we did in class) and an annotated (provide a name and a message with
-m
).Determine how many of the tags in the course website are annotated vs lightweight using. (You may need to use
git pull --tags
in your clone of the course website)
# Tags
<!-- short defintion/description in your own words of what a tag is and what it is for -->
## Comparing tag types
<!-- include your experiment terminal history and interpretation -->
## Inspecting tags
Course website tags by type:
- annoted:
- lightweight:
<!-- include lists of tags for each type -->
12.10. Experience Report Evidence#
12.11. Questions After Today’s Class#
12.11.2. Is the stash diffrent between all the branches or it the same.#
There is one stash per repo, it is a queue shared across branches
12.11.3. with git stash can I create a file on say main stash and then switch to another branch to move that file to that new branch instead?#
Yes, sort of, you can create a while while main is checked out and then stash it instead of committing it. Since it gets stashed, not committed, the file is not “on” the branch.
THen you can switch branches and apply or pop from stash and then commit.
12.11.4. Is the point of stash area just in case you do work in the wrong branch?#
No, it actually cannot help if you commit to the wron branch and if you do not commit, you do not need it.
It is so that you can save work without commiting, get a clean working directory to do something else and then add the work in .
12.11.5. while debugging and you accidently call a good commit a bad commit is there a way to reverse that or no?#
It looks like you would have to start over, but you could print the log first to remember what you had already figured out.
12.11.6. Where does git stash store its stack, is it a file inside of the .git folder?#
it hashes the content as blobs and stores the pointer to that blob in .git/refs/stash
12.11.7. how often are these commands used in a professional environment#
this is hard to know, a lot of people are git novices, but I want you to have these as tools so you can be more efficient.
Being efficient with your time in a salaried job often means you can work fewer hours total or you can get more work done and earn promotions faster.
12.11.8. why wouldn’t i just make a new branch instead of leaving the changes floating in space?#
One time to use stash is when you realize you started working without pulling. If you make a commit you will have to deal with the divergent branches, but if you stash, pull, then apply, no such problem.
Another case is that in some projects, there are standards to not have too many too small commits, so this is a way to avoid that.